Binary Tree C Code Examples For Job Interviews
- Level Order Traversal in a Binary Tree - JournalDev
- Binary tree c code examples for job interviews interview
- Binary tree c code examples for job interviews 2019
- Binary tree c code examples for job interviews
- Binary search trees (Coding for Interviews) · GitHub
If it is found, then searched node is returned otherwise NULL (i. e. no node) is returned. Function is explained in steps below and code snippet lines are mapped to explanation steps given below. [Lines 47-49] Check first if tree is empty, then return NULL. [Lines 50-51] Check if node value to be searched is equal to root node value, then return node [Lines 52-53] Check if node value to be searched is lesser than root node value, then call search() function recursively with left node [Lines 54-55] Check if node value to be searched is greater than root node value, then call search() function recursively with right node Repeat step 2, 3, 4 for each recursion call of this search function until node to be searched is found. Deletion of binary tree Binary tree is deleted by removing its child nodes and root node. Below is the code snippet for deletion of binary tree. 38 void deltree(node * tree) { 39 if (tree) { 40 deltree(tree->left); 41 deltree(tree->right); 42 free(tree); 43} 44} This function would delete all nodes of binary tree in the manner – left node, right node and root node.
Level Order Traversal in a Binary Tree - JournalDev
- Level Order Traversal in a Binary Tree - JournalDev
- Binary tree c code examples for job interviews list
- Binary Search Trees - C Program ( Source Code and Documentation ) - The Learning Point
- Job vacancies 2015 in mauritius south africa
- Binary Tree Tutorials | Interview Questions and Answers
- Oman Air Careers - Latest Vacancies | Regularjobz
- Binary tree c code examples for job interview sur le site
- Binary tree c code examples for job interviews in python
- Allentown area school district job opening song
Binary tree c code examples for job interviews interview
We'll build our trees from arrays that store nodes in what's known as level order. This just means that all of the nodes for a given level of the tree will be adjacent in the input array. This will make more sense if we take an example: [ 1, 2, 3, 4, 5, 6, 7] This input array would correspond to the following tree: How can we turn this array into the tree above, given the tree class we defined earlier? The first thing to notice about the input array is the pattern that it follows: The left child of the node at i will be i * 2 + 1 The right child of the node at i will be i * 2 + 2 Let's write a buildTree function step by step. If we used a for loop to build tree nodes, it might look something like the following. function buildTree ( items) { let root = new Tree ( items [ 0]); for ( let i = 1; i < items. length; i ++) { let node = new Tree ( items [ i]);} return root;} Although this would produce tree nodes for each our array items, there's a pretty big problem here. None of the nodes have their left or right children populated.
Binary tree c code examples for job interviews 2019
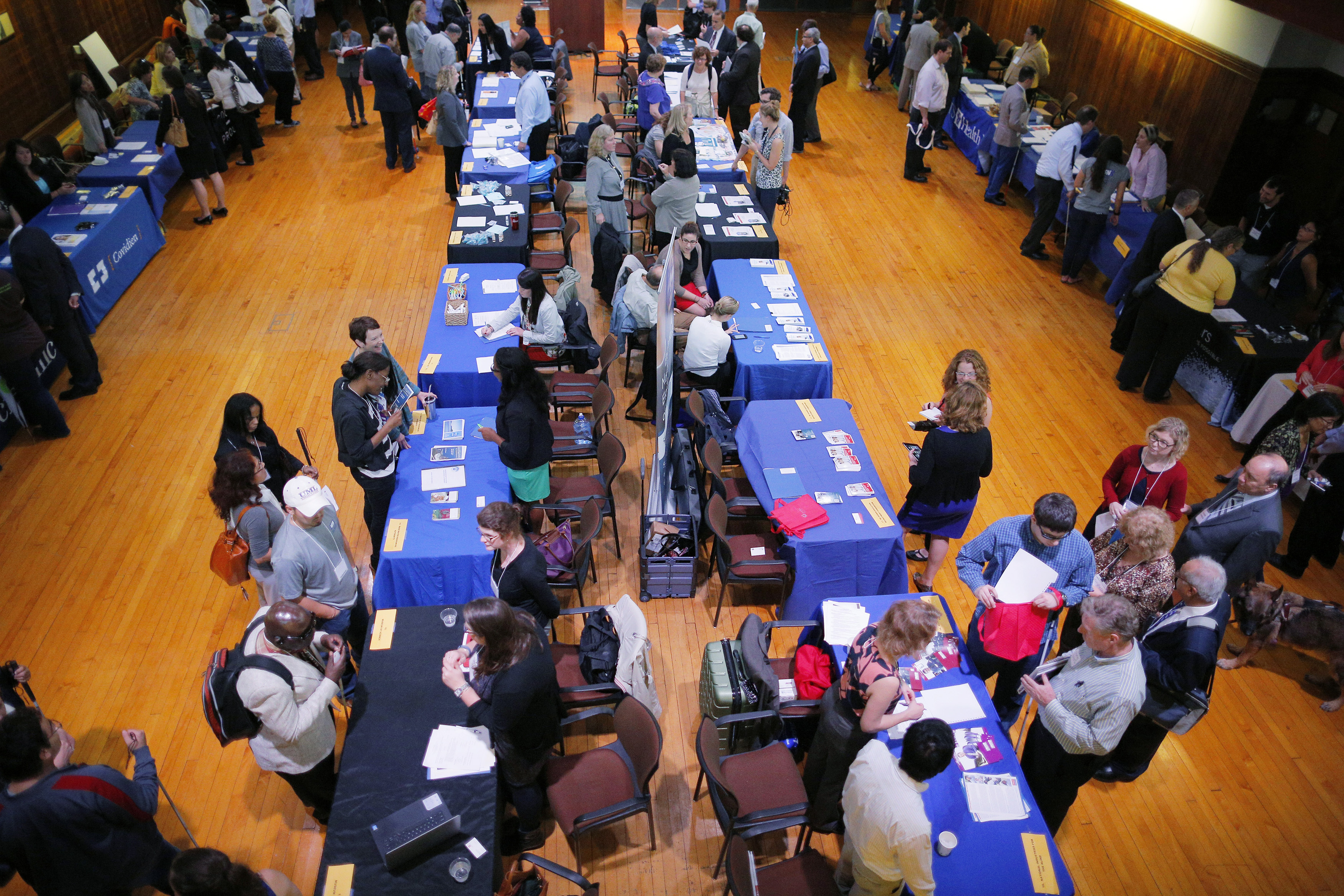
Binary tree c code examples for job interviews
This reduces the complexity of this function pretty noticeably at essentially no cost 1. Print As @Jamal already pointed out, your print function doesn't modify the tree, so it should be const. I'd also prefer to see it allow the user to pass a specific stream to which the contents will be printed. In fact, it's at least worth considering overloading the stream insertion operator ( operator<<) for your tree type, so you can insert a tree in a stream just like you would any other type: Tree my_tree; // code to insert data into tree goes here std::cout << my_tree; Alternative to Print I'd at least consider whether you really want a Tree::print at all, or whether it's better to have the ability to walk the tree and apply essentially any function to all its nodes (with print as just one of those possibilities). Return value from insert I would say that yes, it's a good idea for insert to return a value indicating whether the insertion was successful. Your code is roughly analogous to std::set, which does return such a value, and I've used that return value quite a few times, so if I were going to use this, I'd probably make use of the same.
Binary search trees (Coding for Interviews) · GitHub
What do you think happens when we run this code? void test()
vector
An easier solution would be to use a breadth-first search of the tree. Breadth-first search traverses the tree in level order, which is exactly what we need. In the example above we want our function to return 6. Let's take a look at the code. function bottomLeft ( root) { let nodes = [ root, null]; firstNode = null; while ( nodes. length) { let node = nodes. shift (); if ( nodes. length && node === null) { nodes. push ( null); // End of tree row, insert null to mark new row firstNode = null;} else if ( node) { if (! firstNode) { firstNode = node; // Encountered first node of current row} if ( node. left) { nodes. push ( node. left);} if ( node. right) { nodes. right);}}} return firstNode. data;} console. log ( bottomLeft ( buildTree ([ 1, 2, 3, null, null, 6, 7]))); This is a fairly standard breadth-first search, but there are a few extra quirks specific to solving this problem. Null values in the queue are used to determine where one row of the tree begins and another ends. This is important because the firstNode variable keeps track of the first node in each row, and we wouldn't know when to reset firstNode without some kind of separator value.
C++ Binary Tree Code Examples Overview C++ Binary Tree Code Examples can offer you many choices to save money thanks to 19 active results. You can get the best discount of up to 50% off. The new discount codes are constantly updated on Couponxoo. The latest ones are on Mar 21, 2021 10 new C++ Binary Tree Code Examples results have been found in the last 90 days, which means that every 9, a new C++ Binary Tree Code Examples result is figured out. As Couponxoo's tracking, online shoppers can recently get a save of 50% on average by using our coupons for shopping at C++ Binary Tree Code Examples. This is easily done with searching on Couponxoo's Box. Tips to save money with C++ Binary Tree Code Examples offer You can hunt for discount codes on many events such as Flash Sale, Occasion like Halloween, Back to School, Christmas, Back Friday, Cyber Monday, …which you can get the best discounts. If you buy regularly at a store, do not hesitate to contact us, CouponXoo will support you with an exclusive discount code.
a) Infix Notation (Inorder traversal of a expression tree) b) Postfix Notation (Postorder traversal of a expression tree) c) Prefix Notation (Preorder traversal of a expression tree) A b and c B Only b C a, b and c D None of them Binary Trees Discuss it What are the main applications of tree data structure? 1) Manipulate hierarchical data 2) Make information easy to search (see tree traversal). 3) Manipulate sorted lists of data 4) Router algorithms 5) Form of a multi-stage decision-making, like Chess Game. 6) As a workflow for compositing digital images for visual effects A 1, 2, 3, 4 and 6 B 1, 2, 3, 4 and 5 C 1, 3, 4, 5 and 6 D 1, 2, 3, 4, 5 and 6 Binary Trees Discuss it Level of a node is distance from root to that node. For example, level of root is 1 and levels of left and right children of root is 2. The maximum number of nodes on level i of a binary tree is In the following answers, the operator '^' indicates power. A 2^(i-1) B 2^i C 2^(i+1) D 2^[(i+1)/2] Binary Trees Discuss it Question 4 Explanation: Number of nodes of binary tree will be maximum only when tree is full complete, therefore answer is 2^(i)-1 So, option (A) is true.